Adding a custom case activity to your case Maven-style
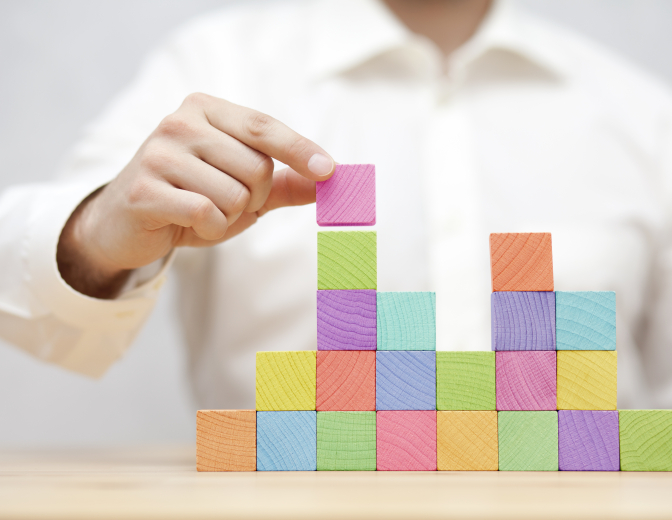
In this blog I’ll show you how to add a simple custom case activity to the case we’ve developed in my earlier blogpost here. I’ll update the GitHub code so that it will reflect the changes I made in the course of this writing.
CustomActivity
ProjectAl right. Let’s get started. First we’ll add a new Java project to our application that will contain our custom java class upon which we’ll base our custom activity. Choose Create Project From Archetype en select org.apache.maven.archetypes:maven-archetype-quickstart as said Maven archetype.
This will give us a new Java project called CustomActivities with a testproject called CustomActivities-Test as an added bonus. If you haven’t done so already, now would be a good time to update JDeveloper and install the JUnit integration extension.
The CustomActivities project contains a simple pom.xml file and a Java file called App.java. Let’s delete this file and its corresponding test file AppTest.java first.
Next add the new project to the aggregate pom, so we can build the whole application with one Maven command.
<?xml version="1.0" encoding="UTF-8"?>
<project xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"
xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<modelVersion>4.0.0</modelVersion>
<groupId>nl.whitehorses.acm</groupId>
<artifactId>CustomActivitiesDemo</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>pom</packaging>
<modules>
<!-- Add project modules -->
<module>CustomActivities</module>
<module>CaseWithCustomActivities</module>
</modules>
</project>
Dependencies
Now to create the custom activity class implementation. We need two libraries, i.e. bpm-services-12.1.3-0-0.jar and oracle.bpm.casemgmt.interface-12.1.3-0-0.jar. The first one is available in the Maven repository we filled with the oracle Maven sync plugin in our previous blogpost. The second library unfortunately is not present at the moment. As a matter of fact none of the acm libraries are synced. I want to stick to Maven so let’s install the second jar manually in our Maven repository. The logical way to store it, is as artifact com.oracle.soa:oracle.bpm.casemgmt.interface. When Oracle adds it to their sync plugin, we can always switch to the groupId:artifactId they choose to store it under. Use the following command to install the jar (change the OFM_HOME to your Oracle Fusion Middleware home directory):
mvn install:install-file -DgroupId=com.oracle.soa -Dversion=12.1.3-0-0 -Dpackaging=jar -DgeneratePom=true -DartifactId=oracle.bpm.casemgmt.interface -Dfile=<OFM_HOME>/soa/soa/modules/oracle.bpm.runtime_11.1.1/oracle.bpm.casemgmt.interface.jar
This will install the library in your local Maven repository:
Now hat we have both libraries in our local repository, let’s add them to our project pom file as depencies. An easy way to do this is by selecting the pom file in JDeveloper, clicking on the plus-icon in the Overview tab and choosing the Add From Repository option. If you miss the newly added case management library, don’t panic. Go to Tools>Preferences>Maven>Repositories and click on the Index Local Repostory icon in the upper right corner . Now you can search for both dependencies and select them. They then will be added to the pom file and the dependencies section will look like this:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.oracle.soa</groupId>
<artifactId>bpm-services</artifactId>
<version>12.1.3-0-0</version>
<scope>compile</scope>
<type>jar</type>
</dependency>
<dependency>
<groupId>com.oracle.soa</groupId>
<artifactId>oracle.bpm.casemgmt.interface</artifactId>
<version>12.1.3-0-0</version>
<scope>compile</scope>
<type>jar</type>
</dependency>
</dependencies>
Custom activity implementation
Lets keep things simple for now. I’ll build a very basic custom activity, just to demonstrate the concept. In a future blogpost I’ll dive a little deeper into the implementation. For now I’ll use the same implementation Danilo Schmiedel used in his blogpost here:
package nl.whitehorses.acm;
import java.util.Map;
import oracle.bpel.services.bpm.common.IBPMContext;
import oracle.bpm.casemgmt.CaseIdentifier;
import oracle.bpm.casemgmt.caseactivity.ICaseActivityCallback;
public class CustomCaseActivity implements ICaseActivityCallback {
public CustomCaseActivity() {
super();
}
public String initiate(IBPMContext iBPMContext,
CaseIdentifier caseIdentifier, String string,
Map<String, Object> map) {
// Add activity logic here
return "Called class CustomCaseActivity for activity => " + string;
}
}
Building the project
Let’s try to build this project first before we move on to the case implementation. Move to the directory of the project POM and type:
mvn clean install
Unfortunately you’ll get the following eror: error: generics are not supported in -source 1.3. Let’s fix this by altering the java compiler version. Add the following snippet to the plugin section of your Maven pom file:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>
1.6
</source>
<target>
1.6
</target>
</configuration>
</plugin>
Now rebuild the project and all should be well.
CaseWithCustomActivities
Now let’s add a custom activity to our case and see if we can initiate it successfully. We’ll make some changes to the case we build in my previous blogpost here.
The caseLet’s first add the custom activity to the case and some other necessary ingredients to test the beasty.
Add a stakeholder Stakeholder and add user weblogic as a member so we can test the custom activity later on with the default case gui.
Next add the custom activity (New > Custom Case Activity) to the case:
Finally add a business rule that activates the (manual) activity upon creation of the case:
This is by far the trickiest part. For the case to be able to call the java implementation, the jar has to be available in the SCA-INF/lib directory. I hoped it was as simple as putting a runtime dependency in the POM file. But that turned out to be only a small part of the puzzle, albeit a necessary part. So let’s add the dependency to the POM first:
<dependency>
<groupId>nl.whitehorses.acm</groupId>
<artifactId>CustomActivities</artifactId>
<version>1.0-SNAPSHOT</version>
<scope>runtime</scope>
<type>jar</type>
</dependency>
Unfortunately this in itself doesn’t do much. The library isn’t included in the project after a Maven build. So we need a way to copy the library from the Maven repostitory into the SCA-INF/lib folder. Luckily we can use the Maven Dependency Plugin for this. Add the following snippet to your pom file.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>2.4</version>
<executions>
<execution>
<id>copy-dependencies</id>
<phase>prepare-package</phase>
<goals>
<goal>copy-dependencies</goal>
</goals>
<configuration>
<includeScope>runtime</includeScope>
<outputDirectory>${project.basedir}/SOA/SCA-INF/lib</outputDirectory>
<overWriteReleases>false</overWriteReleases>
<overWriteSnapshots>true</overWriteSnapshots>
<excludeTransitive>true</excludeTransitive>
</configuration>
</execution>
</executions>
</plugin>
The plugin is tied to the prepare-package phase (right before the actual sca gets packaged) and by adding the includeScope=runtime configuration parameter we make sure only runtime dependecies get copied into the project.
Now for neatness let’s use the Maven Clean Plugin to clean up the added dependecies. Add the following snippet:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-clean-plugin</artifactId>
<version>2.5</version>
<configuration>
<filesets>
<fileset>
<directory>${project.basedir}/SOA/SCA-INF/lib</directory>
<includes>
<include>**/*</include>
</includes>
</fileset>
</filesets>
</configuration>
</plugin>
Testing it all
Now that all the hard work is done. Let’s install the whole application and give it a testdrive:
mvn clean install -Duser=weblogic -Dpassword=<password>
After the case is deployed, we can test it with SoapUI:
Next open the Case gui (http://localhost:7101/bpm/workspace) and check the case we’ve just created. The CustomCaseActivity has been activated and can be launched right now.
Finally click the CustomCaseActivity, enter a comment and click OK. After a short while you should see a message stating that the instance was initiated successfully.
Conclusion
With a little help of a few Maven plugins we’ve built an application consisting of a case project with a custom case activity and a java project containing the custom case activity implementation. We’ve deployed the application via Maven and have seen it succesfully in action. I think the addition of Maven is one of my personal favorites out of the 12c new features section. Bye bye custom made Ant scripts! With Maven we now have a default versioning system for our services and java libraries and we can benefit from dependency management out of the box. Continuous Integration has become a whole lot easier to achieve in 12c.
The application code can be found on GitHub here.
References- Create a custom Case Activity in Oracle BPM Case Management
- How to Create a Custom Case Activity
- Apache Maven Compiler Plugin
- Apache Maven Dependency Plugin
- Apache Maven Clean Plugin
- Github code